Thinking & coding: the Navigation homescreen
Today I start programming my app. The language is Dart and the Framework is Flutter. Based on my design, I first looked up which widgets are interesting for my app.
You can find my design here.
If you want to help, there 's a comment section in this post. Thank you in advance.
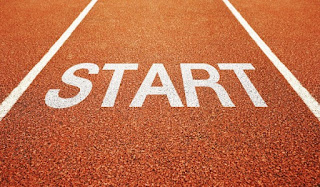
The thoughts
The Scaffold widget is the backbone of my screen. And we are going to build on that. Now I immediately ask myself whether I should place my background design in the scaffold or later in a screen. Now according to the documentation, the Scaffold has no decoration option, so later I will have to use a widget that does have a decoration option.
The next widget from which I looked up the documents is the "Navigation Rail". From the documentation I have taken the following important things. The Navigation Rail is the first part of a Row () so it is on the left side. And this Row is high in the widget tree. And this is again in a Scaffold () body. And if I want to keep the rail as narrow as possible, as in my prototype, i can not us the labels. I can also give an elevation which creates a depth. Instead of a divider.
The second widget will be added to the Row () next to the navigation rail. And this widget should be my selected screen. Now the question is when I make a screen, how much space will it take up. How do I determine the size.
Routing is done via the NavigationRailDestination, where you need at least 2 of them. I'm going to use 5 of them. These would then place my screens.
I want to give the rail a background color and use my icons from my Figma prototype.
Code
So I have written the code for the NavigatieRail. Just tinkered with the setstate, to link the icons in the rail to and index. I did not know where in the code to declare the "selectedIndex". But now everything is working properly. After the navigation is linkt to the screens, i want to extract the screen code in to a special screens map after.
I have used a Statfull widget for the home screen because a have to setstate to the index.
next step is to get the custom icons in to my navigationbar,
Do, believe and be happy,
Stefaan
A big thanks to:
Flutter explained and all community members.
The growing developer
Reso coder
Tadas Petra
In the future i will connect this blog with the Github repository.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( home: HomeScreen(), ); } } class HomeScreen extends StatefulWidget { HomeScreen({Key key}) : super(key: key); @override _HomeScreenState createState() => _HomeScreenState(); } class _HomeScreenState extends State<HomeScreen> { int selectedIndex = 0; @override Widget build(BuildContext context) { return Scaffold( body: Row( children: [ NavigationRail( onDestinationSelected: (newIndex) { setState(() { selectedIndex = newIndex; }); }, elevation: 1, backgroundColor: Colors.green[100], groupAlignment: 0, selectedIndex: selectedIndex, destinations: [ NavigationRailDestination(icon: Icon(Icons.home), label: Text('Home')), NavigationRailDestination(icon: Icon(Icons.view_agenda), label: Text('Events')), NavigationRailDestination(icon: Icon(Icons.local_parking), label: Text('Parking')), NavigationRailDestination(icon: Icon(Icons.fastfood), label: Text('Food')), NavigationRailDestination(icon: Icon(Icons.help), label: Text('S.O.S.')), ], ), Expanded( child: Container(), ) ], ), ); } } |
Comments
Post a Comment